Lab 1: Dot Displayer
Please see the README.md file in the project for required intermediate deliverables.
Learning Outcomes
- Demonstrate competency in many prerequisite areas including:
- Graphical User Interfaces with JavaFX
- Text file input
- Handling exceptions in a robust and meaningful fashion
Resources Needed
- Video introduction
- If you need to install Java, please follow these instructions.
- Accept the GitHub Classroom assignment invitation in Canvas and then, in IntelliJ, create a new project from Version Control using the repository URL. If you haven't used this before, see the pre-lab activity that was part of CS1011 Lab 6.
- If you haven't created an executable JavaFX
jar
file before, this Self-Learning Module from CS1021 should help. - Be sure that CheckStyle is installed and configured. If you are not familiar with CheckStyle or need to reconfigure it, this Self-Learning Module from CS1011 should help.
Overview
Prior to smartphones and tablets, parents resorted to flattened wood pulp and sticks of colored wax to occupy their kids' attention. One popular App for paper and crayons was called "Connect the Dots" or "Dot to Dot." The "screen" consisted of a collection of numbered dots. The user was required to draw lines between consecutive dots. If the user worked the App correctly, a piece of art emerged. In this assignment you will build the foundation for a multi-week project. The subsequent two laboratory assignments will build on this one, allowing you to improve your initial submission, based on feedback from your instructor, and expand the program's functionality.
Assignment
You must create a program that will read a picture from a .dot
file
and graphically display the picture stored in the file.
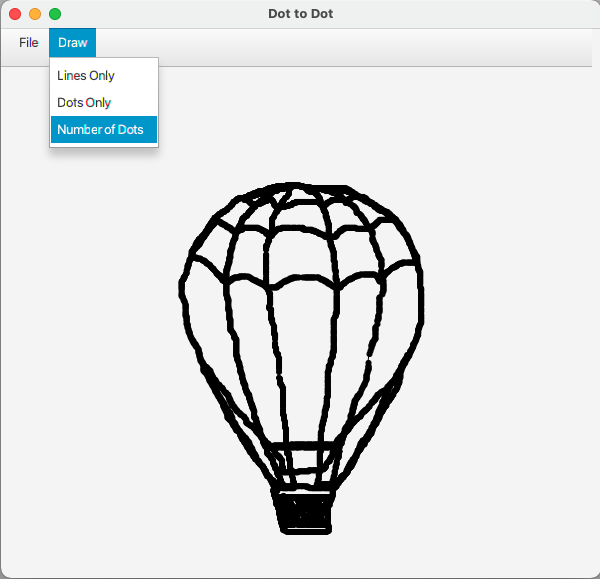
Your program must have two menu items:
- File
- Open — Loads a
.dot
file and displays the dots in the file. In addition, it connects neighboring dots with lines (including connecting the last dot back to the first dot). - Close — Exits the program (use
Platform.exit()
)
- Open — Loads a
- Draw
- Lines Only — Redraws the loaded picture drawing only lines (not dots).
- Dots Only — Redraws the loaded picture drawing only dots (not lines).
Note that the Number of Dots menu item is not required for this lab but will be an added requirement in lab 2.
The Draw menu items should only be active when a picture has been loaded.
Class Structure
All of your classes should be placed in a package whose name matches your MSOE username.
You must implement two classes:
Dot
— Represents a dot in the picture.Picture
— Holds a list ofDots
that describe a picture.
The Picture
class must implement the following methods:
void load(Path path)
— Loads all of the dots from a.dot
file.void drawDots(Canvas canvas)
— Draws each dot in the picture onto the canvas.void drawLines(Canvas canvas)
— Draws lines between all neighboring dots in the picture on the canvas.
.dot
File Format
Your program must be able to read dot files that contain a list of dots.
The file is formatted such that each dot is on a separate line. A dot
consists of a horizontal and vertical component separated by a comma.
Each component is a floating point value between zero and one. For
example, square.dot
looks like this:
0.1, 0.1
0.1,0.9
0.9,0.9
0.9, 0.1
There are several .dot
files in the data
folder for the repository.
Drawing Dots
A dot can be drawn on a Canvas
by first obtaining the GraphicsContext
and then calling the fillOval()
method. Be sure to set an appropriate
fill color and center the dot over its coordinate location.
Drawing Lines
Lines should be drawn between all neighboring dots, including a line
from the last dot back to the first dot. The lines can be drawn on a
Canvas
by first obtaining the GraphicsContext
and then creating a
path. To do this:
- Call
beginPath()
- Move to the location of the first dot by calling
moveTo()
- Draw a line to the next dot by calling
lineTo()
- Repeat the previous step for all dots
- Call
closePath()
- Call
stroke()
to draw the path
Exception Handling
There are several situations that could cause your program to throw
an exception. For example, if the file is not found, cannot be opened,
or contains incorrectly formatted data, it is likely that an exception
will be thrown. In these cases, the program should display an useful
message in an Alert
.
Just For Fun
Ambitious students may wish to:
- Try replacing
lineTo()
withquadraticCurveTo()
orbezierCurveTo()
. - Add number annotations next to each dot.
- Create a print to PDF option.
Acknowledgment
This laboratory assignment was developed by Dr. Chris Taylor.