CSC1110 - Week 12 Exercise
Overview
In this exercise you will gain practice with inheritance, interfaces, and enums.
Resources
- Accept the GitHub Classroom assignment invitation in Canvas and then, in IntelliJ, create a new project from Version Control using the repository URL.
Polymorphism
Inheritance allows us to define relationships between different classes and reuse code that behaves identically across the classes. One of the benefits of Java's Object Oriented Programming (OOP) approach regarding inheritance is the concept of Polymorphism. Poly (multiple) morph(shape) ism(ism) is the idea that a single entity can have multiple shapes. In the case of Java and OOP, it means we can have an instance of a class that can also be defined as an instance of a different class or interface.
The way this occurs is when a class either implements an Interface or extends a parent class, the type of that class can be identified as both the class type itself and the type of the interface or parent class. Take, for example, the ArrayList class. When an ArrayList
is initialized, it can be stored in either an ArrayList
variable or a List
variable, which is one of the interfaces that ArrayList
implements. What this allows us to do is define methods and variables that are identical in form and use to other implementing or extending classes in a single location, the parent class or interface.
A Deck of Cards
Most of us are familiar with a standard deck of playing cards. 52 cards in 4 suits (Clubs, Diamons, Hearts, Spades) of 13 cards each. But there are many card games that use variations of a standard deck, and thus the makeup of the deck will be different for different games. The game Pinochle, for example, uses 48 cards: the same 4 suits, but two copies of each card from 9 to Ace (9, 10, J, Q, K, A). But the basic actions and functions of the decks are the same. We will shuffle the deck, deal cards from the deck, add cards to the deck, etc. So by building a parent Deck
class, we can extend the class and make some child classes that will inherit all that funtionality, and only change the data and methods that would be unique to those classes.
The Abstract class
An abstract class is like any other class we write with one exception: it has at least one abstract method
. Recall that all methods other than default
methods in an interface are abstract and must be implemented by the class that implements the interface. The same is true for an abstract
class. Any class that extends the abstract
class must implement the abstract
methods contained in the parent class.
The Deck
class
The Deck
class will be an abstract class that will have only a single abstract method: addCard(). This rest of the methods will be normal, implemented methods. A few things to note about the Deck
instance methods
- drawCard() will remove a card from the top of the deck (i.e. the front of the list)
- shuffle(), contains(), and toString() will use prebuilt methods in the
Collections
andArrayList
classes.
The Card
class
A playing card has two pieces of information: its rank and its suit. When comparing two cards, we will use the provided compareTo() method that is required by the Comparable
interface. A Card
is equal to another when both the rank and suit of the cards match.
The Poker Deck
A Poker deck is a standard 52 card deck that contains one of each card from 2 to A in all four suits. When adding a card to the deck, the addCard method must make sure that are currently less than 52 Card
s in the Deck
and that the Card
being added does not yet exist in the Deck
. Otherwise, the method should simply not add the Card
The Euchre Deck
A Euchre deck is different from a Poker deck only in that it does not have cards of a rank lower than 9, so only cards from 9 to A in all four suits, meaning it will only contain 24 cards. When adding a Card
to this Deck
, the addCard method must check that there is not yet 24 Card
s in the Deck
, that the Card
being added does not yet exist in the Deck
and that the Card
is at least of rank 9.
The Exercise
Using the information above, the UML diagram below, and the provided code, fully implement the incomplete classes.
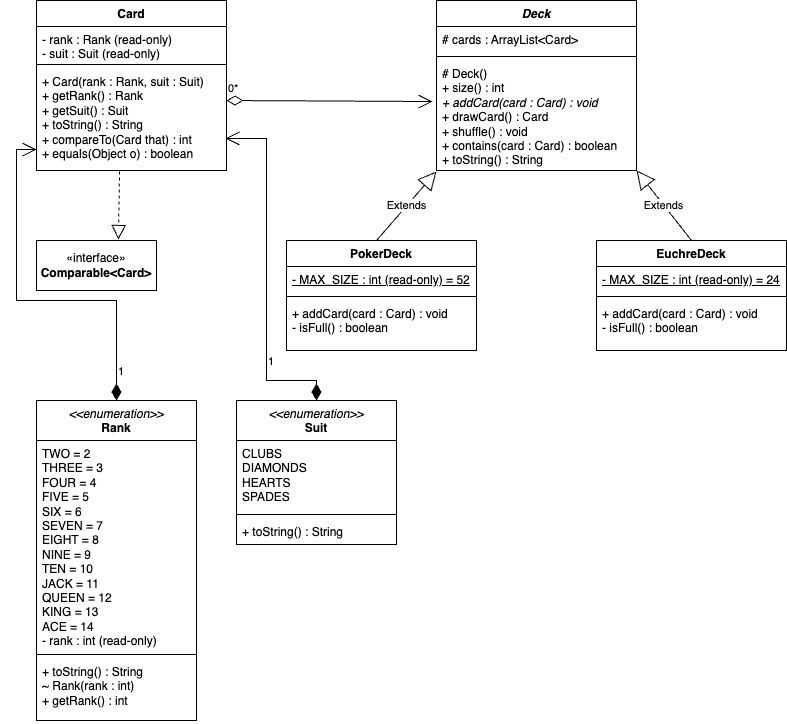
Submission Instructions:
Commit and push your code to your GitHUb repository.